In this article we are going to look at how we can alter a form and insert a custom function to get called when the form gets submitted. In Drupal 7, one or multiple callback functions get called when the user presses the submit button, you know, to process it. In between you can also have validation functions - as you can see best on node add/edit forms - but I invite you to read this article for more info on how to add your own validation callback to an existing form.
So to exemplify how to add a custom callback, let’s debug an existing form and see what’s in there. Let’s use hook_form_alter() to do this, so throw the following code into your custom module, making sure you have the Devel module installed first:
function your_module_form_alter(&$form, &$form_state, $form_id) {
dpm($form);
}
Clear your caches and go edit a node or add a new one. You’ll notice inside the $form
array of the debug information 2 elements (array themselves) called #validate
and #submit
.
The first one contains validation callbacks and the second one, you guessed it, the submit callbacks. On this form there is no submit callback because it uses a button level handler and the callback is therefore defined in here:
$form['actions']['submit']['#submit']
You’ll have to navigate in the debug information and you’ll find the node_form_submit()
function:
Now let’s look at another example. Edit any existing block on the site and try to locate the #validate
and #submit
elements of the $form
array. You’ll notice that here the callback function is found at the higher level. And yet another good reason why when developing in Drupal, debugging with Devel is such a great help.
Having set a bit of the context, let’s get back to the job at hand. How do we make sure our own custom functionality gets called when the form is submitted? We have to insert our own callback function somewhere in there. But first, let’s create it:
function custom_callback() {
drupal_set_message('This is my custom message');
}
Above we have a simple function that uses drupal_set_message()
to output a string in the usual Drupal place. We’d like therefore this message to be printed after the user presses the submit button on our form. How crazy a?
As you’ve seen, there are 2 places where you can insert your custom callback function - at the button level or at the higher form level. Let’s go with the node edit form and insert it at the higher level first and see what happens:
function your_module_form_alter(&$form, &$form_state, $form_id) {
if ($form['#form_id'] == 'article_node_form') {
$form['#submit'][] = 'custom_callback';
}
}
Here we target the node edit form of the article content type and insert our custom callback at the higher level. Now if you edit a node, you should see the custom message get printed out onto the page next to the usual node edit message. One thing to notice is that it gets printed out first, before the regular one. That means it gets called before the button level handler. So if we want it to be called after, we’d add it there:
function your_module_form_alter(&$form, &$form_state, $form_id) {
if ($form['#form_id'] == 'article_node_form') {
$form['actions']['submit']['#submit'][] = 'custom_callback';
}
}
Since the function name is the second one in the array of functions, it gets called second and our message gets printed out after the normal node processing takes place. Similarly, on the block configuration form, all you have to do is add your callback in the right order of functions you want called. And that’s pretty much how you can hook into the Drupal Form API and do a bunch of cool stuff. Like redirecting to another page after form submission.
Hope this helps.
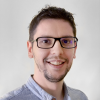
Daniel Sipos
Danny founded WEBOMELETTE in 2012 as a passion project, mostly writing about Drupal problems he faced day to day, as well as about new technologies and things that he thought other developers would find useful. Now he now manages a team of developers and designers, delivering quality products that make businesses successful.
Comments
Callback Function
Useful information to add a custom callback function to an existing form in Drupal.
How to push file from Drupal to Alfreso using custom module
i have to upload multiple documents from Drupal to Alfresco repository and tract the same as reports in Drupal. for that i have used CMIS module to integrate with Drupal.
To make all these happen, i have to follow below steps:
I would be really helpful if i get solutions for above in any method..even,
Thanks in advance :)
custom call back function not called
This is not working
$form['actions']['submit']['#submit'][] = 'custom_callback';
watchdog in callback
Do not put watchdog function into the callback function. "Server has gone away" message will be shown
this helps
yep this very helpfull
Add new comment