In this tutorial I will show you how your module can define its own permission and the proper API way of checking whether a user has a certain permission or not.
First of all, let’s see how we define the permission and let Drupal know of its existence. It is actually not very complicated. We use hook_permission() for that. Like usual, first the code, then the explanation:
function demo_permission() {
return array(
'my demo module permission' => array(
'title' => t('Administer my Demo module'),
'description' => t('Allow people to make use of my module.'),
),
);
}
This hook returns an associative array which contains another array the key of which being the machine name of the permission (the same name we will be checking against later). Inside this array, you define the title and description of the permission, i.e. the text that will appear on the admin/people/permissions page.
After adding this code into your module (changing of course demo with the name of your module and defining a more descriptive permission name), clear your cache and go to that page I mentioned above. You’ll see that you can assign now this permission to your user roles. The administrator role has by default checked this permission.
OK, so you have defined your permission, but you have not achieved anything unless you also restrict certain actions in your module to user roles that have this permission. There are multiple ways of doing this. If you implement hook_menu() for instance, you pass the permission name as an access argument to the menu item access callback.
However, there are other cases in which you need to check whether a user account has a certain permission. There is a Drupal API function well suited for this purpose, called user_access(). You pass the permission and the user account object and the function will return TRUE if the user has that permission.
If you don't have the user account object on hand, it will automatically use the logged-in user account, which is basically what you want in most cases to check against.
So let’s see it in action:
function demo_node_view($node, $view_mode, $langcode) {
if(user_access('my demo module permission')) {
drupal_set_message('Test');
}
}
In this example I use hook_node_view() that gets called on each node view and I check if the logged-in user has the permission we just defined. If they do, Drupal outputs a message on the screen using drupal_set_message(). Pretty simple.
So that’s pretty much it. Drop a comment if you have any questions.
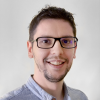
Daniel Sipos
Danny founded WEBOMELETTE in 2012 as a passion project, mostly writing about Drupal problems he faced day to day, as well as about new technologies and things that he thought other developers would find useful. Now he now manages a team of developers and designers, delivering quality products that make businesses successful.
Comments
If you want to define a custom rule for a permission?
Hi, I need to define a custom rule for a permission only for a user and for a node (not a permission for a role), for example , I have hook_permission() with a new permission named "acces to publications" , but now I need to define a custom rule for it. An example: Some user is refered by reference in a node, and I want to assign to this user (not to his role) a permission for post commments, ¿Is it possible?. Sorry for my crap english.
Best Regards !
In reply to If you want to define a custom rule for a permission? by R0ber (not verified)
User?
Why not just make a role and have only that one user in it?
Users can't have permissions
As far as I know.
What you could do is look for the current user in the user entity reference list for the node and if they are not in that list, hide the post comments form.
Add new comment