Have you ever used hook_node_access() to control access to various node operations on your site? Have you found this hook unbelievably awesome? Thought to yourself, boy, I'm unstoppable now? Well, I sure did.
The problem is that Drupal 7 entities do not start and end with nodes and you may want to control access to other entities in the same way. However, there is no hook_taxonomy_term_access()
or hook_fieldable_panels_pane_access()
that you can use to apply this tactic. So what can you do in these cases?
The first thing is to understand that each entity implementation is different. Some have a centralised access callback for all the operations while others simply define permissions or access callbacks to be used directly inside the hook_menu()
definition. And I'm sure there are also other ways of handling this but these I think are the most common.
In this article we are going to look at two entity examples: taxonomy terms (from core) and fieldable panels panes (from contrib). We will trace the access pipeline from request to response and see what we can do to intervene in this process and add our own logic. So let's begin.
Taxonomy terms
The first entity type we are going to look at is the regular taxonomy term introduced by core. It's actually pretty easy to understand how this entity type is built and what we can do in order to hook into the access pipeline.
If we take a look at taxonomy_menu(), we can quickly figure out which paths it creates to add and edit terms and what access callback and arguments are defined:
Adding terms to a vocabulary
$items['admin/structure/taxonomy/%taxonomy_vocabulary_machine_name/add'] = array(
'title' => 'Add term',
'page callback' => 'drupal_get_form',
'page arguments' => array('taxonomy_form_term', array(), 3),
'access arguments' => array('administer taxonomy'),
'type' => MENU_LOCAL_ACTION,
'file' => 'taxonomy.admin.inc',
);
Editing a particular term
$items['taxonomy/term/%taxonomy_term/edit'] = array(
'title' => 'Edit',
'page callback' => 'drupal_get_form',
// Pass a NULL argument to ensure that additional path components are not
// passed to taxonomy_form_term() as the vocabulary machine name argument.
'page arguments' => array('taxonomy_form_term', 2, NULL),
'access callback' => 'taxonomy_term_edit_access',
'access arguments' => array(2),
'type' => MENU_LOCAL_TASK,
'weight' => 10,
'file' => 'taxonomy.admin.inc',
);
By nature, viewing taxonomy term pages has to do with node access since they show a list of nodes so we will skip this aspect here and stick with just the add/edit operations.
So let's change the access callbacks and potential passed arguments inside a hook_menu_alter() implementation.
/**
* Implements hook_menu_alter().
*/
function demo_menu_alter(&$items) {
$items['admin/structure/taxonomy/%taxonomy_vocabulary_machine_name/add']['access callback'] = 'demo_taxonomy_term_access';
$items['admin/structure/taxonomy/%taxonomy_vocabulary_machine_name/add']['access arguments'] = array(3, 'add');
$items['taxonomy/term/%taxonomy_term/edit']['access callback'] = 'demo_taxonomy_term_access';
$items['taxonomy/term/%taxonomy_term/edit']['access arguments'][] = 'edit';
}
So what happens here? We are simply replacing the access callback definition for these two menu items with our own custom function demo_taxonomy_term_access()
. Additionally, we standardise a bit the arguments this function gets: $entity
(either term or vocabulary object) and $op
(add or edit). This covers both cases.
Now let's write our callback function:
/**
* Access callback for taxonomy term add/edit operations
*
* @param null $entity
* @param null $op
* @return bool
*/
function demo_taxonomy_term_access($entity = null, $op = null) {
if ($op === 'add') {
return user_access('administer taxonomy');
}
if ($op === 'edit') {
return taxonomy_term_edit_access($entity);
}
}
Inside the function we run a check on $op
and perform the access logic we want. In doing so, we make use of the $entity
object that can be either the term being edited or the vocabulary to which a new term is added. In this example we are replicating exactly the intended access checks of the Taxonomy module. To add a term, a user needs to have the administer taxonomy
permission while to edit one it needs either the same permission or a vocabulary specific permission (as seen inside taxonomy_term_edit_access()). Now it's up to you to include inside this logic whatever else you need. And you end up with something not so dissimilar to hook_node_access()
but for taxonomy terms.
Fieldable Panels Panes (FPP)
FPP is a contributed module that creates an entity type that is primarily used inside a Panels context. Regardless of any of this though, it too exposes CRUD operations on the entities of this type. And consequently, there are access implications. So let's see how we can hook into this pipeline by starting where we did with the Taxonomy module: at fieldable_panels_panes_menu()
.
By looking inside this hook implementation we can find many defined paths which relate to these CRUD operations. And we also see that many of them have fieldable_panels_panes_access()
as the access callback with some specific arguments passed to it.
But what does this function actually do? Nothing but taking the parameters and deferring to the controller class responsible for this entity type and its access()
method. And by checking fieldable_panels_panes_entity_info()
, the principle function responsible for defining the entity type, we find that this is the PanelsPaneController
class. In there, we find all the logic for determining access rights for various operations.
Now that we know all this, what can we do to hook into this pipeline? We could do like before and override the hook_menu()
implementation. But since there are so many menu items and the FPP class controller is already doing such a nice job, that may be counter productive. So let's instead override the entity definition and replace the class controller with one of ours that extends PanelsPaneController
. In there, we then do what we want.
First, we implement hook_entity_info_alter():
/**
* Implements hook_entity_info_alter().
*/
function demo_entity_info_alter(&$entity_info) {
$entity_info['fieldable_panels_pane']['controller class'] = 'DemoPanelsPaneController';
}
Right after this, we create a file inside our module called DemoPanelsPaneController.inc
which contains the following to start with:
<?php
/**
* Overrides fieldable panels panes controller functionality
*/
class DemoPanelsPaneController extends PanelsPaneController {
}
Finally, we edit the module's .info
file and make sure it loads this file:
files[] = DemoPanelsPaneController.inc
Then we clear the cache. If all went well, nothing really has changed on the site in terms of functionality. However, the DemoPanelsPaneController
class is being used for controlling the fieldable_panels_pane
entity type. And since this one extends PanelsPaneController
, all previous functionality remains. It follows to now override the access()
method and include our own logic to it:
public function access($op, $entity = NULL, $account = NULL) {
// $account not always full (defaults to current user)
return parent::access($op, $entity, $account);
}
In the example above, nothing is really changed because the logic is deferred back to the parent class. But you could add some logic in addition or instead of that depending on various contextual factors.
However, I strongly recommend/warn you to stick to the minimum amount of deviation from default is needed, and for all rest, defer back to the original logic. This is to prevent the opening of any security holes. For example, if your access()
method looks like this:
public function access($op, $entity = NULL, $account = NULL) {
if ($op === 'update') {
return false;
}
}
You are indeed denying access to the edit form but now anybody can create and delete entities because there is no more check on those operations. So make sure you understand this and do not leave any loopholes. The fix in this case would be:
public function access($op, $entity = NULL, $account = NULL) {
if ($op === 'update') {
return false;
}
return parent::access($op, $entity, $account);
}
If the operation is edit, the access is denied for everybody (probably not a good idea but suitable for this demo purpose). However, if the operation is delete
, create
or view
, we defer to the logic of the parent class to handle those cases. In which case the default FPP permissions will be used.
Conclusion
In this article we've seen two ways we can hook into the access checking pipeline of entities in Drupal 7. We've learned that there is more than just one way of going about it depending on how the entity type in question has been defined. The purpose was to illustrate how you can approach the matter and where you need to look in order to find a solution. Hope this helps.
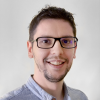
Daniel Sipos
Danny founded WEBOMELETTE in 2012 as a passion project, mostly writing about Drupal problems he faced day to day, as well as about new technologies and things that he thought other developers would find useful. Now he now manages a team of developers and designers, delivering quality products that make businesses successful.
Add new comment