In this article I am going to talk a bit about a solution to a problem I encountered while developing an application with CodeIgniter. I was building a small photo management system which needed CRUD (create-read-update-delete) operations. And whenever the user was to perform such an operation, I wanted to let him/her know that the operation was completed (or an error had occurred).
A very common practice in ensuring a good user experience is returning the user back to the form after the submission was processed. This is the case especially for administration forms and not necessarily for survey-like forms for which a dedicated confirmation page also makes sense. In addition, the user expects the redirect to be accompanied by a status message confirming or disconfirming the success of his/her operation.
The problem
In CodeIgniter, when you process a form submission at the same path as the one showing the form, you can easily output the confirmation message. In the respective Controller method, before loading the form View, you can check for and process the POST data and depending on the result, pass along a confirmation message in a variable. As a result, the user experiences a page reload but with a status message regarding the operation s/he performed.
But what do you do in more complicated situations in which you have to submit the form to another path (be it a different method in the same Controller or another Controller altogether)? You are basically redirecting the user to a completely different page which most likely is not used for displaying any content. So you either need to load the form again there or redirect him/her back to the original form.
A “bad” way of doing this is loading the form in this method as well. This would mean repeating code and you end up having 2 urls for the same form. And what do you do if you have multiple forms you need processed by this same Controller method? Luckily, CodeIgniter comes equipped with all you need for the “good” way.
The solution
So if loading the form again is “bad”, redirecting back to the old form is the alternative. The CodeIgniter URL Helper comes to the rescue with the redirect()
function that performs a header redirect to the path you specify as a parameter.
redirect('/login/form/', 'refresh');
Just make sure you load the URL Helper prior to expecting this to work.
So now the form is processed in another Controller and the user is redirected back. But how to pass along the confirmation message?
After some pondering, I found the solution for this problem in the CodeIgniter session class. There is this thing called flashdata which is basically session data that persists only for one server request, being cleared right after. Intrigued are you? I was too.
CodeIgniter supports this technique and it is perfect for our need. It is also very easy to use. First, make sure you have the session class loaded of course. Second, in the Controller that does the form processing, store the confirmation message as new flashdata:
$this->session->set_flashdata('data_name', 'data_value');
Third, in the Controller that displays the form (to which you redirect back), check to see if there is any flashdata and retrieve it like so:
$message = $this->session->flashdata('data_name');
You can then pass $message
along to the View for being displayed above the form. And that’s it.
User submits the form, the form gets processed by another Controller method, the latter stores the message in the session flashdata and redirects the user to the original Controller method that loads the form. However, the latter Controller also checks if there is any flashdata set to display above the form. If there is, it will appear only that one request since flashdata won’t be present a second time.
By the by, if you need this flashdata to persist for one more server request, you can use the keep_flashdata()
function.
Pretty cool. Thank you CodeIgniter. Hope this helps someone.
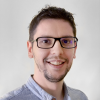
Daniel Sipos
Danny founded WEBOMELETTE in 2012 as a passion project, mostly writing about Drupal problems he faced day to day, as well as about new technologies and things that he thought other developers would find useful. Now he now manages a team of developers and designers, delivering quality products that make businesses successful.
Comments
Thankyou
thankyou Danny it's very helpful
Thanks a lot.
Thanks a lot.
teting, but its limited by the cookie
the data passed are limited by the cookie 4k size, this due flash data ues the session for that.. its no so standar but works in mayor cases..
Very helpful
Thank you bro. It worked perfectly fine
For multi dimensional arrays
Wow...passing data to view in CodeIgniter is quite easy. Also, if you have multi dimensional arrays, you can generate multiple loops by creating loops. Source: https://www.cloudways.com/blog/how-to-pass-data-in-codeigniter/
Thank you very much!
It works very well :)
Great
Thank you i was facing the same problem actually !
Thanks a lot
i need help for redirect and return whith post data.
Thanks dear for this solution. but I have a problem. when I make a post on a form that redirects me to another page and in this page I still make another form post, when I use the back button of my browser I have an error saying "The document has expired ". I have to refresh the page for the data to be loaded. I really need help finding a solution. Thank you Best regards.
Keep alive issue
keep_flashdata() when we use this error error message keep duplication on each request...
Add new comment